A GridView is there with multiple rows binded to it from database.You want a functionality to navigate to a new page when user clicks on a Coressponding Control placed on each GridView Row.
How to achieve this?
Use a HyperLink Field as shown below:
<asp:HyperLink ID="HyperLink4" runat="server" ToolTip='Click To Edit' ImageUrl="pen.png" NavigateUrl="<%# String.Format("javascript:void(window.open('../Dec1/EditNewTemp.aspx?tempid={0}'),null,'width=525,height=450,top=325,left=2000,scrollbars=yes,resizeable=no');", Eval("tempid")) %>" > </asp:HyperLink>
Aliter:Write the code as below in RowDataBound event of GridView to pass required value to a
Java Script Function.
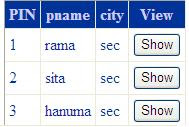
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowIndex == -1) return;
Button B = (Button)e.Row.FindControl("btn1");
B.Attributes.Add("onclick", "JavaScript:fun1('" + DataBinder.Eval(e.Row.DataItem, "pinno") + "')");
}
Note: btn1 is the ID of Button control in GridView. When we click on this Button , the corresponding rows PrimaryKey Column value should be passed to a JavaScript function.
The javacript function is as below:
function fun1(id)
{
window.open("Default2.aspx?pinno="+id,"New","width=400,height=200,left=0,top=100");
}
Our requirement is to watch results in a different page which requires a QueryString
value to execute its query.
When user clicks on 'Show' Button corresponding to PinNo=3 ,the Result will be as shown below :
Aliter 2 : Opening a New Window using Response.Redirect:<asp:TemplateField HeaderText="View">
<ItemTemplate>
<asp:ImageButton CommandName="View" ID="View" ToolTip='Click To View' runat="server" CommandArgument='<%# Eval("tempid") %>' ImageUrl="view.gif" Width="20" Height="20" OnClientClick="form1.target ='_blank';"/>
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" />
</asp:TemplateField>
Note : form1 is the ID of the Form.You need to change this to yours Form ID in order to observe the
change in functionality.
The code will be written in RowCommand Event of GridView as shown below:
protected void VideoListGrid_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "View")
{
string tid = e.CommandArgument.ToString();
Session["tid"] = tid.ToString();
Response.Redirect("../Dec8/NewTempAPI.aspx?tempid="+tid);
}
}
Suppose that your GridView contains other buttons which need to be opened on the same window.
Then What should we do?
Simply include this code part in the source code for Button.
OnClientClick="form1.target ='_self';"